APIs using the OpenAPI Specification and AutoRest along with Azure API Management
This blog post will look at how the OpenAPI Specification can be used with Azure API Management and AutoRest to create feature-rich APIs. Throughout this post, we will cover the following points:
- What are APIs?
- OpenAPI Specification
- Azure API Management
- AutoRest to auto generate code
- How can we use the Open API Specification and Azure API Management to write the API implementations in using AutoRest and VS Code
What are APIs
An application programming interface, or API, is an interface that can be used between multiple pieces of software to communicate. It defines what type of calls or requests can be made, how to use them, and the data types. Building an API aims to abstract the underlying implementation and expose what is needed by the developer.
To look at a straightforward example, we can consider an application that displays data help in a database. Say an ordering system, for example. The application will need to insert orders into the database when customers place them. Customers will also want to view their orders, so retrieving data from the database would be required. The application could contain code to select and insert the data, but the developer would need to know about the structure of the tables and data. That tightly couples the application to the database though. What happens if other systems in the same company need the same data? They too would have to write their implementations.
In the above example, an API would remove the developer’s need to know details about the database and make it re-usable across multiple systems.
API’s can also be created for public consumption. Let’s say that you want to retrieve flight arrivals into a particular airport. That in itself seems like a complicated requirement. However, there is an open-source API provide by OpenSky Network. From the documentation, we can see an operation that will give what we are looking for and documentation on the parameters for the request and response, along with the data types.Using a popular tool such as Postman, we can get arrivals into London Heathrow on the 16thJanuary 2021, with no code written.
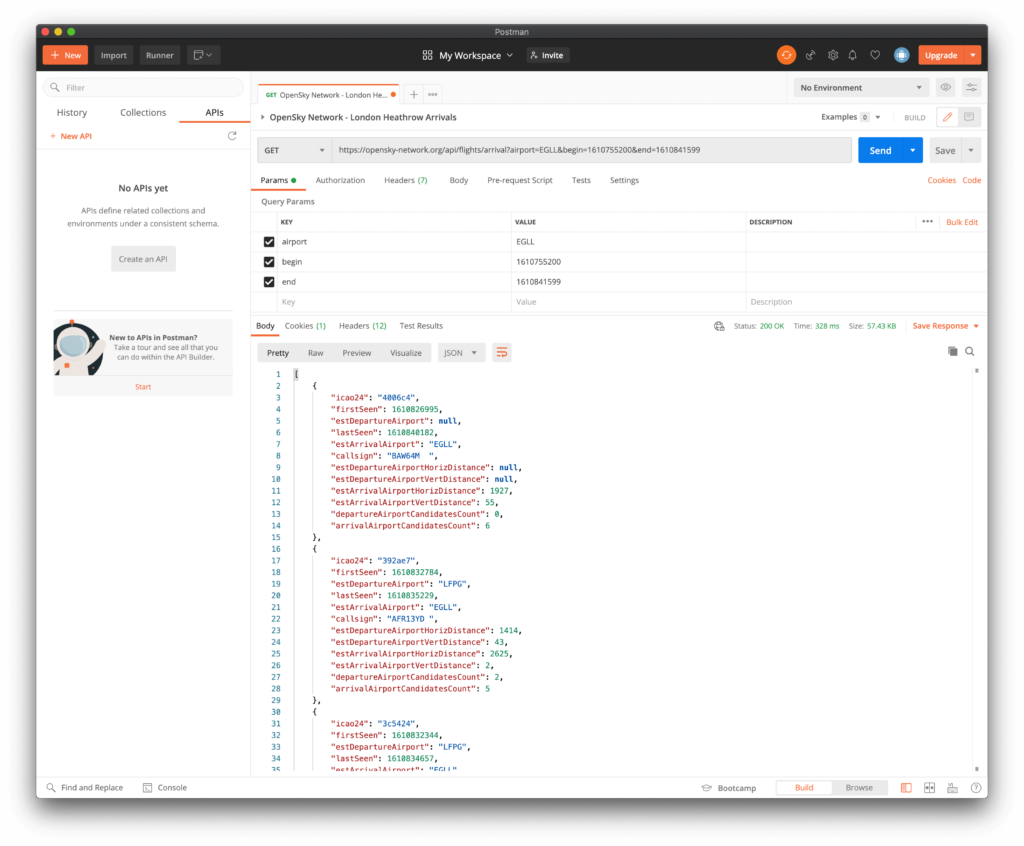
With everyone writing API’s, though, how do you manage standards? If your organisation has a lot of, how are they maintained? These two questions can be answered by using the OpenAPI Specification and Azure API Management.
OpenAPI Specification
The first question is “What is the OpenAPI Specification?”. To answer this, we can take a look at the description on the Swagger site:
The OpenAPI Specification (OAS) defines a standard, language-agnostic interface to RESTful APIs which allows both humans and computers to discover and understand the service’s capabilities without access to source code, documentation, or through network traffic inspection. When properly defined, a consumer can understand and interact with the remote service with minimal implementation logic.
An OpenAPI definition can then be used by documentation generation tools to display the API, code generation tools to generate servers and clients in various programming languages, testing tools, and many other use cases.
https://swagger.io/specification/
So we have a language-agnostic interface which can allow us to create re-usable and documented RESTful APIs for consumption.
With API architecture accelerating in growth, more and more people and organisations are defining APIs. When it comes to streamlining this process, Azure API Management is a perfect tool within the enterprise.
API Management
API Management allows the management of API architecture in a single place. It can do many things, such as managing APIs across clouds. Help protect resources by applying call rates, for example, saving development work and standardising. What is interesting for this blog post, though, is the ability to define APIs and export the OpenAPI Specification. Rather than talk about it, let’s create an API definition.
First of all, lets set up the resources required:
GROUPNAME=api-management-example
LOCATION=<LOCATION>
SERVICENAME=BlogPosts
PUBLISHEREMAIL=<EMAIL ADDRESS>
PUBLISHERNAME=<COMPANY NAME>
az group create --name ${GROUPNAME} --location ${LOCATION}
az apim create --name ${SERVICENAME} --resource-group ${GROUPNAME} --location ${LOCATION} --sku-name Consumption --enable-client-certificate true --publisher-email ${PUBLISHEREMAIL} --publisher-name ${PUBLISHERNAME}
Once the API Management resource has been created, let’s create an API. To make this demo a bit easier, we will use an OpenAPI Specification made for this blog posts. It’s not complete, but it serves the purpose.
Before you proceed, download the API specification from this site using the following link:
Blog Posts OpenAPI Specification – YAML 1.35 KB 28 downloads
YAML file that is used in the ‘Serverless Azure Functions using API Management and…- Open your API Management resource
- In the left navigation of your API Management instance, select ‘APIs’.
- Select ‘Add API’
- Select the OpenAPI tile.
- Enter the values from the following table. Then select Create to create your API.
Setting | Value |
---|---|
OpenAPI specification | Select the location of the file downloaded above. |
Display name | After you enter the preceding service URL, API Management fills out this field based on the JSON. |
Name | After you enter the preceding service URL, API Management fills out this field based on the JSON. |
API URL suffix | blog |
If all goes well, you should see something like this:
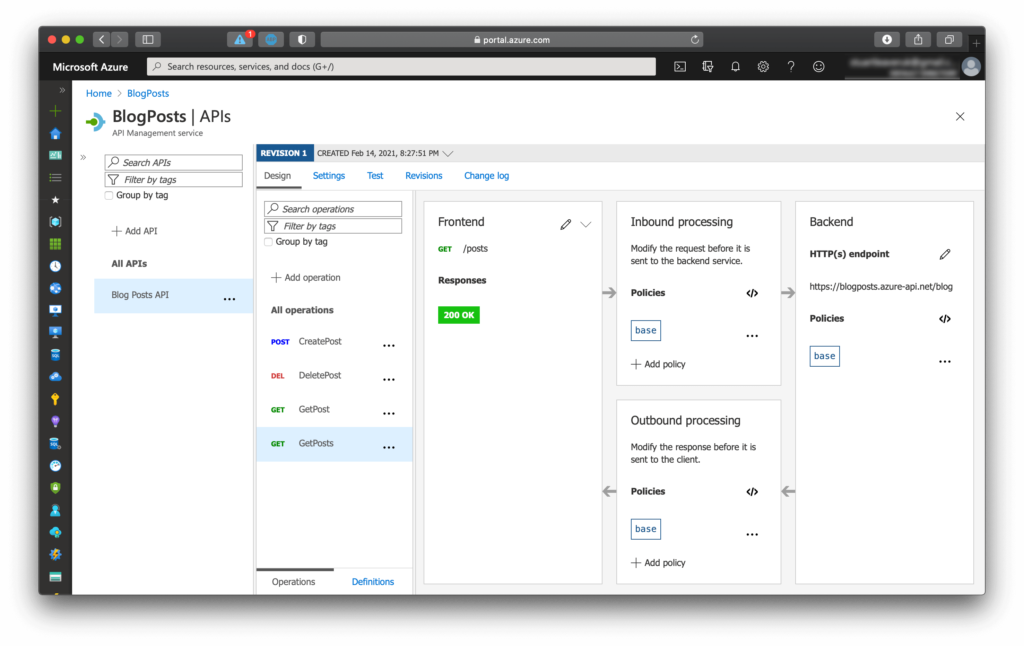
Let’s assume for this post that the OpenAPI Specification just imported, was written by someone other than a developer. A developer may now have the implement the API, so how can the process be made easier? We can use AutoRest.
AutoRest
AutoRest is a tool that generates client libraries for accessing RESTful web services, but how can we use it to help us out.
First of all, make sure you have AutoRest installed. This can be done using the following commands. You’ll see that the package is installed globally.
npm install -g autorest
autorest –latest
In the folder where the YAML file is that you downloaded earlier, run the command below. You can use the following values for the language flag:
Language | Description |
---|---|
–-python | Python |
–-csharp | C# / .NET code |
–-java | Java |
–-typescript | Typescript |
–-go | Golang |
autorest --input-file=blog-posts.openapi.yaml --v3 –-csharp
There are other flags you can set, such as –output-folder and –namespace. You can find a full list here or a common list here.
Once the code generation has run, if you open the folder in VS Code, you can see the generated code, and for example, see the GetPosts method:
/// <summary> Get blog post by ID. </summary>
/// <param name="id"> Format - int32. </param>
/// <param name="cancellationToken"> The cancellation token to use. </param>
public Response GetPost(int id, CancellationToken cancellationToken = default)
{
using var message = CreateGetPostRequest(id);
_pipeline.Send(message, cancellationToken);
switch (message.Response.Status)
{
case 200:
return message.Response;
default:
throw _clientDiagnostics.CreateRequestFailedException(message.Response);
}
}
From here, you can start adding the rest of your implementation and in this case, getting data around blog posts.
Summary
In this post, we have looked at the OpenAPI Specification, Azure API Management and AutoRest.
The OpenAPI Specification is language-agnostic and can define the operations and data types used in APIs. As a result of API architecture becoming a popular way of writing systems, it has become necessary to manage them. So rather than having multiple teams writing their own API’s you could have a tool such as Azure API management to do the work for you.
Azure API Management allows the creation of rich API’s that can be managed in one place. It can also take away the need for developers to implement logic such as call rate limits and usage quotas, for example. However, a developer may still be required to implement these APIs in code.
This is where AutoRest comes in. The APIs defined in an API Management resource can be exported in the OpenAPI standard. AutoRest can then take this file and autogenerate the code the developer needs to get started.Hopefully, this post gives you a better understanding of how the OpenAPI Specification, along with Azure API Management and AutoRest can help generate APIs. If you would like more information, there are some useful links below.
Links
OpenAPI Specification (About) – https://swagger.io/specification/
OpenAPI Specification – http://spec.openapis.org/oas/v3.0.3
Azure API Management – https://azure.microsoft.com/en-gb/services/api-management/
AutoRest – https://github.com/Azure/autorest